Sorting Arrays in Swift
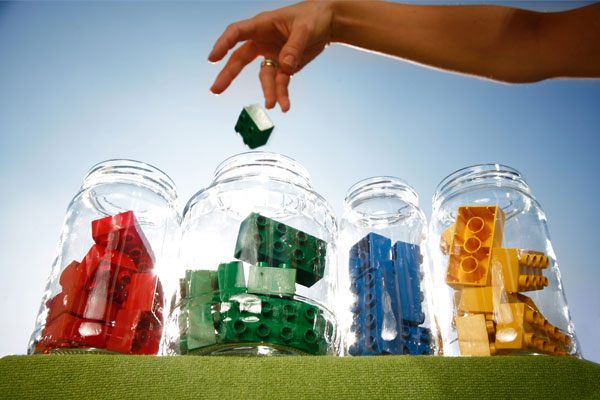
Sorting arrays is a very common task. Swift has a built-in feature that is fast and easy to use. In this article you’ll learn all the details.
Hint: This post is using Swift 3
sort and sorted
Imagine we have an array of integers:
var intArray = [34,13,14,584,1,34,69,3,596,2]
If the members of the array have a type that confirms to the Comparable protocol, then you can use the function sorted() to get an ordered array. Int does confirm to Comparable, so let’s do it:
let result = intArray.sorted() print(result) //[1, 2, 3, 13, 14, 34, 34, 69, 584, 596]
So, that was very easy! Alternatively, we can also use the function sort(). In contrast to sorted(), it doesn’t return a new array but modifies the existing one. Of course this is only possible if the array is not a constant.
intArray.sort() print(intArray) //[1, 2, 3, 13, 14, 34, 34, 69, 584, 596]
However, not all types confirm to the Comparable protocol.
Sorting arrays of dates
A good example for a type that doesn’t confirm to the Comparable protocol is Date. So if we want to sort an array of Date, then we can use
public func sorted(by areInIncreasingOrder: (Element, Element) -> Bool) -> [Element]
or
public mutating func sort(by areInIncreasingOrder: (Element, Element) -> Bool)
The only difference to the functions in the previous paragraph is, that we have to hand over a closure that has two array elements as parameters and returns a boolean. If the two elements are already in the right order, we have to return true. Sounds very straightforward. Let’s take a look at an example:
var dateArray = [Date]() let dateFormatter = DateFormatter() dateFormatter.dateFormat = "MM/dd/yyyy" dateArray.append(dateFormatter.date(from:"02/03/1985")!) dateArray.append(dateFormatter.date(from: "12/01/2025")!) dateArray.append(dateFormatter.date(from: "07/08/1964")!) dateArray.append(dateFormatter.date(from: "09/04/2016")!) dateArray.append(dateFormatter.date(from: "01/01/2000")!) dateArray.append(dateFormatter.date(from: "12/12/1903")!) dateArray.append(dateFormatter.date(from: "04/23/2222")!) dateArray.append(dateFormatter.date(from: "08/06/1957")!) dateArray.append(dateFormatter.date(from: "11/11/1911")!) dateArray.append(dateFormatter.date(from: "02/05/1961")!) dateArray.sort { (date1, date2) -> Bool in return date1.compare(date2) == ComparisonResult.orderedAscending } for date in dateArray { print(dateFormatter.string(from: date)) }
And indeed, the result is as expected:
Performance
How is the performance? Is the build-in algorithm a good choice for sorting arrays? Yes, it is! As you can see in this article, a self implemented sort algorithm could be quicker in some situations, but generally speaking the performance is very good.
Differences between Swift 2 and Swift 3
It’s worth to mention that there’s a difference between Swift 2 and Swift 3. All the examples in this post are written in Swift 3. In Swift 2, the functions have different names.
- Swift 2: sort() -> Swift 3: sorted()
- Swift 2: sortInPlace() -> Swift 3: sort()
For more details about the changed API naming, you can take a look at this Swift evolution proposal: Apply API Guidelines to the Standard Library.
Video
Check out this video as well:
References
Image: @ S.Pytel / shutterstock.com