Two Useful Log Messages
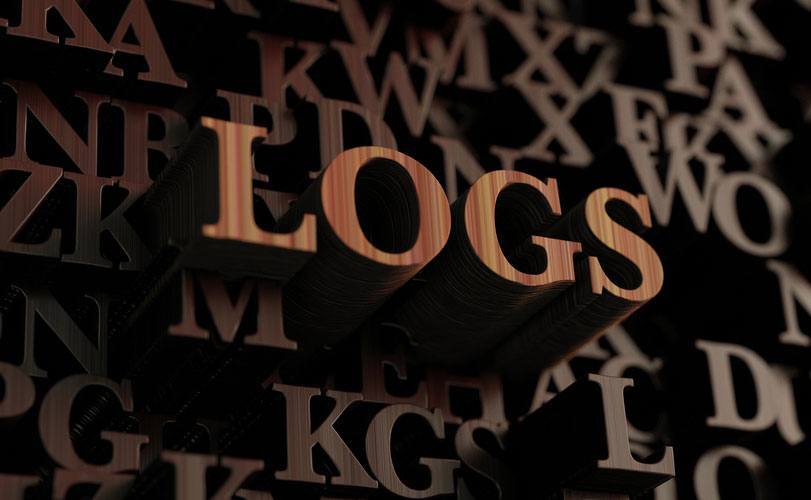
Logging is a very useful method for debugging an app. Most times, though, too much information is logged. But there are some very useful things you should log. Let’s take a look at two of them.
Hint: This post is using Xcode 8.2.1, iOS 10 and Swift 3
Simulator: Detecting App Location
You can debug your app on both the simulator and a real device. Obviously, both methods have their pros and cons. Generally speaking, debugging on a real device is more precise and is the way to go. But sometimes it also good to use the simulator because the deployment is faster and you can easily switch between many different screen sizes. Additionally, you can also access the app directory, which can be very useful.
However, it’s not that easy to actually find the location of the app folder on your Mac. The easiest way it so simply print the location to the console after the app has been launched. Just insert the following code into the didFinishLaunchingWithOptions method of your app delegate:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool { if let url = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first { print(url.path) } return true }
Now copy that location from your console, open your finder, open the “go-to” dialogue by pressing shift-cmd-g, and paste that location.
Detecting Memory Leaks
Thanks to the automatic reference counting (ARC), you don’t have to deal a lot with memory management. However, it’s still possible to produce memory leaks. This can happen, for example, if you have a so-called retain cycles. A very common scenario for that is that a view controller and its delegate have strong references to each other. After the view controller disappears from the screen, it won’t be deallocated because of that scenario. This will increase the memory usage of your app and, eventually, your app could be terminated by the operating system.
A very easy and good approach for testing whether a view controller gets deallocated properly or not, is by just putting a log message inside its deinit method:
deinit { print("second viewcontroller deinit") }
In certain situations the view controller should be deallocated, for example after popping it from the navigation controller. You can check that by looking at the console because the deinit will only be called, if the view controller really gets deallocated.
For more details about retain cycles and techniques to detect them, take a look at my blog post Retain Cycles, Weak and Unowned in Swift.
Video
References
Title image: @ Christopher Titze / shutterstock.com