UserDefaults
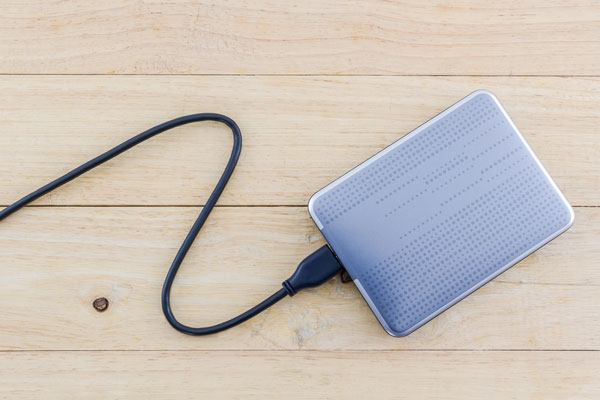
UserDefaults are a great way to persist a small amount of data. In this post we will discuss all the things you need to know.
Hint: This post is using Swift 3 and iOS 10
There are several ways to persist data on iOS. You can use for example Core Data or write a file to the filesystem and each way has its use cases. You should use UserDefaults for a small amount of data like some settings the user chooses in the app. The data persists across reboots of the app. For an overview about all the different ways to persist data on iOS, take a look at this post: Data Persistence On iOS – An Overview.
What kind of data can be saved?
Essentially, these types can be saved:
- String
- Data
- Number
- Date
- Array
- Dictionary
It’s important to note that arrays and dictionaries have to be property lists so that they can be saved by using UserDefaults. Arrays and dictionaries are property lists, if they only store values of the types mentioned above.
Based on these types, there are other types that can be saved. Internally they will be converted to one of the types already mentioned:
- Int
- Float
- Double
- Bool
- URL
So for example an Int will be converted to Number automatically.
Saving data by using UserDefaults
So how can you save a value to UserDefaults? First, you always need an instance of the UserDefaults. You can access it by using the property standard:
UserDefaults.standard
Now you can use one of the set methods. For each value you want to save, you have to specify a key:
UserDefaults.standard.set(true, forKey: "useDarkTheme")
So it’s behaving very much like a dictionary.
Reading data by using NSUserDefaults
Accessing your saved data is also very straightforward:
let useDarkTheme = UserDefaults.standard.bool(forKey: "useDarkTheme")
If there is no boolean saved yet for that key, false will be returned. Other functions like
func string(forKey defaultName: String) -> String?
return nil instead.
Synchronize
It used to be good practice to use the method synchronize() in certain scenarios. However, this method has become deprecated, so you shouldn’t use it anymore.
Best practices
The usage of UserDefaults is a little bit cumbersome in real projects, so it’s good practice to create a special class for managing all UserDefaults values:
class UserDefaultsManager { private static let useDarkThemeKey = "useDarkThemeKey" static var useDarkTheme: Bool { get { return UserDefaults.standard.bool(forKey: useDarkThemeKey) } set { UserDefaults.standard.set(newValue, forKey: useDarkThemeKey) } } }
In this example we are using computed properties to handle the read and write operations. By using this approach, it’s very comfortable to access the value:
UserDefaultsManager.useDarkTheme = true print(UserDefaultsManager.useDarkTheme)
References
Image: @ iceink / shutterstock.com